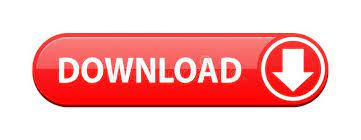

The following example simplifies this code by instantiating the Action delegate instead of explicitly defining a new delegate and assigning a named method to it. Private Sub ShowWindowsMessage(message As String) MessageTarget = AddressOf Console.WriteLine MessageTarget = AddressOf ShowWindowsMessage If Environment.GetCommandLineArgs().Length > 1 Then If Environment.GetCommandLineArgs().Length > 1 thenĭelegate Sub DisplayMessage(message As String) Type DisplayMessage = delegate of message: string -> unit Private static void ShowWindowsMessage(string message) If (Environment.GetCommandLineArgs().Length > 1) MessageTarget = gcnew DisplayMessage(&Console::WriteLine) ĭelegate void DisplayMessage(string message) MessageTarget = gcnew DisplayMessage(&TestCustomDelegate::ShowWindowsMessage) If (Environment::GetCommandLineArgs()->Length > 1) Static void ShowWindowsMessage(String^ message) Public delegate void DisplayMessage(String^ message) For example, the following code explicitly declares a delegate named DisplayMessage and assigns a reference to either the WriteLine method or the ShowWindowsMessage method to its delegate instance. When you use the Action delegate, you do not have to explicitly define a delegate that encapsulates a method with a single parameter. To reference a method that has one parameter and returns a value, use the generic Func delegate instead. It can also be a method that returns a value that is ignored.) Typically, such a method is used to perform an operation. In Visual Basic, it must be defined by the Sub… End Sub construct. This means that the encapsulated method must have one parameter that is passed to it by value, and it must not return a value. The encapsulated method must correspond to the method signature that is defined by this delegate. You can use the Action delegate to pass a method as a parameter without explicitly declaring a custom delegate. ' This code will produce output similar to the following:
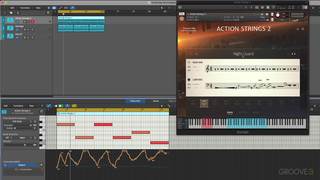
' Display the contents of the list using the Print method. (* This code will produce output similar to the following: The following demonstrates the lambda expression feature of F# Display the contents of the list using the print function. F# provides a type alias for as ResizeArray. * This code will produce output similar to the following: to display the contents of the list to the console. The following demonstrates the anonymous method feature of C# Display the contents of the list using the Print method.

Similarly, in the C# example, an Action delegate is not explicitly instantiated because the signature of the anonymous method matches the signature of the Action delegate that is expected by the List.ForEach method. Instead, it passes a reference to a method that takes a single parameter and that does not return a value to the List.ForEach method, whose single parameter is an Action delegate. Note that the example does not explicitly declare an Action variable.
In addition, the C# example also demonstrates the use of anonymous methods to display the contents to the console. In this example, the Print method is used to display the contents of the list to the console. The following example demonstrates the use of the Action delegate to print the contents of a List object. The parameter of the method that this delegate encapsulates.
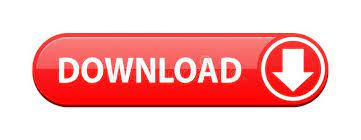